You run into trouble with your scope, The way you use ``tasklist`` is like a global (*not in the Global scope but in the functions scope*) But JSOM continues async so ``tasklist`` gets all different values This will get you on the right track: https://sharepoint.stackexchange.com/questions/164912/query-multiple-lists-using-javascript-csom (and off that oldskool IE8 use of ``createDelegate``) #Update As said you problem is the scope Think of it as your shoppingCart, you dropped items in another You also do **not** have to carry that whole ``this`` scope with you 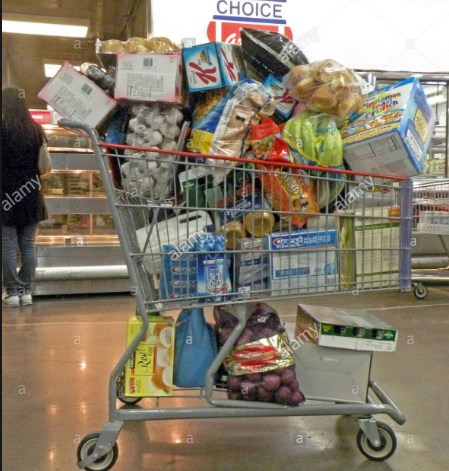 You can use JavaScripts ``.bind()`` to only carry with you (to other functions) what you need: ### Find all Documents Lists in all SubSites (webs): function getProjectSites() { var clientContext = new SP.ClientContext.get_current(); var webCollection = clientContext.get_web().getSubwebsForCurrentUser(null); clientContext.load(webCollection); clientContext.executeQueryAsync(inspectWeb.bind(webCollection), null); } function inspectWeb() { var webCollection = this;//bound by calling definition var webEnumerator = webCollection.getEnumerator(); while (webEnumerator.moveNext()) { var subWeb = webEnumerator.get_current(); var shoppingCart = { sitetitle: subWeb.get_title(), url: subWeb.get_serverRelativeUrl(), list: subWeb.get_lists().getByTitle('Documents') } // this is from your code!! (abusing) a global clientContext // stricty speaking you should get the correct context based on the siteurl again // that way the inspectWeb function could be used (with a url PARAMETER) to inspect // individual Webs. Your function can now only work in the async chain // and only as no other code messes with the ``clientContext`` global var clientContext.load(shoppingCart.list); console.log('inspecting Site: ' + shoppingCart.sitetitle); clientContext.executeQueryAsync( inspectList.bind(shoppingCart),//successCallback function () {// errorCallback (for site without a Document list) console.warn('empty listCollection in', shoppingCart.url); } ); } } function inspectList() { var list = this.list;//var shoppingCart = this; console.log(list.get_itemCount(), 'items in', list.get_title(), 'from', shoppingCart.url); //to get the items itself you have to do more (async) calls: getItems } getProjectSites();